Python Dictionaries
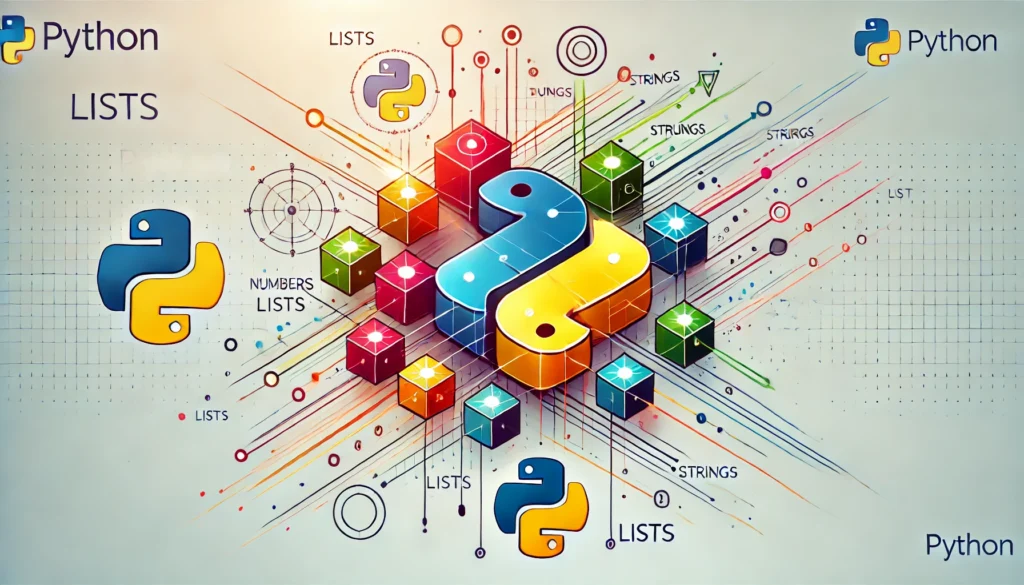
Python is a versatile and widely-used programming language, with various built-in data structures that simplify working with data. One of the most powerful and flexible of these structures is the dictionary. This blog post will delve into what a Python dictionary is, why it’s used, how it differs from other collections, and how you can use its many functions with examples.
What is a Python Dictionary?
A dictionary in Python is an unordered collection of key-value pairs. It allows you to store, retrieve, and manipulate data using a unique key for each value. Unlike lists or tuples, which are indexed by position, dictionaries are indexed by keys. Each key is associated with a value, forming a pair known as a mapping.
In Python, a dictionary is represented using curly braces {}
with key-value pairs separated by colons (:
). For example:
my_dict = {
"name": "Vikram",
"age": 30,
"company": "ITXperts"
}
In this dictionary, "name"
, "age"
, and "company"
are the keys, and "Vikram"
, 30
, and "ITXperts"
are their corresponding values.
Why Use a Dictionary?
Dictionaries are used when you need a fast and efficient way to map keys to values. Some common use cases include:
- Quick lookups: Searching for a value using its key is incredibly efficient, with an average time complexity of O(1).
- Data associations: When you want to associate meaningful identifiers (keys) with values, a dictionary is a natural fit. For example, in a database-like structure, names could be associated with phone numbers or product IDs with descriptions.
- Flexible and dynamic: You can add or remove key-value pairs easily, which makes dictionaries ideal for storing dynamic or changing data.
Differences Between Dictionaries and Other Collections
Python offers several other collection types, such as lists, tuples, and sets, which serve different purposes. Here’s how dictionaries differ from these:
Feature | Dictionary | List | Tuple | Set |
---|---|---|---|---|
Structure | Key-Value pairs | Ordered collection of items | Immutable ordered collection of items | Unordered collection of unique items |
Mutable | Yes | Yes | No | Yes |
Duplicates | Keys must be unique, values can repeat | Allows duplicates | Allows duplicates | No duplicates allowed |
Access Time | O(1) average for lookups by key | O(n) for lookups by index | O(n) for lookups by index | O(1) for membership testing |
Use Case | Mapping relationships between data | Ordered data, simple sequence storage | Ordered immutable sequence | Unique, unordered collection |
Key Differences
- Indexing: Lists and tuples are indexed by position (integer), while dictionaries use unique keys.
- Order: Dictionaries prior to Python 3.7 did not maintain order, but from Python 3.7 onward, they retain insertion order. Sets, on the other hand, are unordered.
- Mutability: Both dictionaries and lists are mutable, but a tuple is immutable (once defined, it cannot be changed).
How to Create a Dictionary
There are multiple ways to create a dictionary in Python:
1. Using Curly Braces
The most common way to create a dictionary is by using curly braces {}
and adding key-value pairs.
employee = {
"name": "John Doe",
"role": "Software Developer",
"age": 28
}
2. Using the dict()
Constructor
The dict()
constructor can be used to create a dictionary.
employee = dict(name="John Doe", role="Software Developer", age=28)
3. Using a List of Tuples
You can also create a dictionary by passing a list of tuples, where each tuple contains a key-value pair.
employee = dict([("name", "John Doe"), ("role", "Software Developer"), ("age", 28)])
Common Dictionary Functions and Methods
Python provides a wide range of functions to interact with dictionaries effectively. Here are some key ones:
1. dict.get(key, default)
Returns the value for a specified key if it exists, otherwise returns the default value.
employee = {"name": "John", "age": 30}
print(employee.get("name")) # Output: John
print(employee.get("role", "Not Assigned")) # Output: Not Assigned
2. dict.keys()
Returns a view object containing all the keys in the dictionary.
employee = {"name": "John", "age": 30}
print(employee.keys()) # Output: dict_keys(['name', 'age'])
3. dict.values()
Returns a view object containing all the values in the dictionary.
print(employee.values()) # Output: dict_values(['John', 30])
4. dict.items()
Returns a view object containing the dictionary’s key-value pairs as tuples.
print(employee.items()) # Output: dict_items([('name', 'John'), ('age', 30)])
5. dict.update(other)
Updates the dictionary with elements from another dictionary or from key-value pairs.
employee.update({"role": "Developer"})
print(employee) # Output: {'name': 'John', 'age': 30, 'role': 'Developer'}
6. dict.pop(key, default)
Removes the specified key and returns the corresponding value. If the key is not found, it returns the default value.
age = employee.pop("age")
print(age) # Output: 30
print(employee) # Output: {'name': 'John', 'role': 'Developer'}
7. dict.clear()
Removes all items from the dictionary, leaving it empty.
employee.clear()
print(employee) # Output: {}
Examples of Using Dictionaries
Example 1: Dictionary as a Database Record
Dictionaries are often used to store records of data where each piece of data is mapped to a descriptive key.
product = {
"id": 101,
"name": "Laptop",
"price": 800,
"stock": 50
}
# Accessing values
print(product["name"]) # Output: Laptop
# Updating stock
product["stock"] -= 1
print(product["stock"]) # Output: 49
Example 2: Storing Multiple Records Using a List of Dictionaries
You can combine lists and dictionaries to store multiple records.
employees = [
{"name": "John", "age": 30, "role": "Developer"},
{"name": "Jane", "age": 25, "role": "Designer"},
{"name": "Doe", "age": 35, "role": "Manager"}
]
# Accessing employee details
for employee in employees:
print(f"{employee['name']} is a {employee['role']}")
Example 3: Counting Frequency of Words Using a Dictionary
Dictionaries can be useful for counting occurrences, like tracking the frequency of words in a text.
text = "apple orange banana apple banana apple"
word_list = text.split()
word_count = {}
for word in word_list:
word_count[word] = word_count.get(word, 0) + 1
print(word_count) # Output: {'apple': 3, 'orange': 1, 'banana': 2}
Conclusion
Python dictionaries are an incredibly powerful tool for mapping relationships between keys and values. They offer fast lookups, flexibility in storage, and a wide range of built-in methods for efficient manipulation. Whether you’re organizing data, performing lookups, or building more complex data structures, dictionaries provide an intuitive and efficient way to achieve your goals.
Understanding how to use Python dictionaries and leveraging their full potential can help you write cleaner, more efficient, and more readable code.
By learning to work with dictionaries, you’ll gain a deeper understanding of Python’s data structures, enabling you to build more efficient and scalable applications.